Introduction
In Java development, bytecode manipulation is an advanced technique used to modify or generate the behavior of classes at runtime. JavaAssist is an open-source bytecode manipulation library that provides a relatively simple way to handle Java bytecode, allowing developers to perform bytecode-level operations in a manner close to Java code. This article will introduce the functions, usage methods, and application scenarios of JavaAssist in detail.
JavaAssist
JavaAssist is an advanced bytecode manipulation tool that provides a relatively simple method for editing and creating Java bytecode. Compared to directly using bytecode manipulation libraries like ASM, JavaAssist makes operations more closely aligned with Java programming itself by providing more advanced APIs. Below, we will delve into the technical principles of JavaAssist, including its working mechanism, core components, and implementation methods.
Working Mechanism
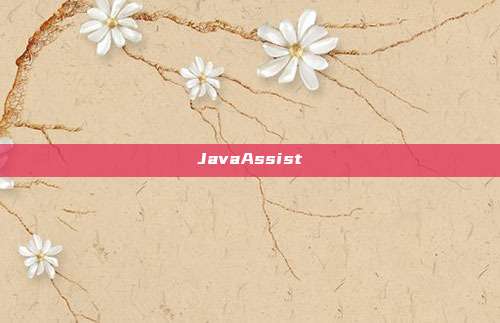
JavaAssist operates at the level of Java class files (.class files), and it can dynamically modify the structure and behavior of classes before or during class loading. JavaAssist mainly works in the following ways:
1. Direct Editing of Class Files:JavaAssist can read existing .class files or generate new class files, and then modify the structure of the class, such as adding or modifying fields, methods, etc., through its API.
2. Dynamic Modification at Class Loading TimeJavaAssist can modify the bytecode of a class when it is loaded into the JVM by defining a class loader or using Java's Instrumentation API.
Core Components
The operations of JavaAssist mainly revolve around the following core components:
1. ClassPoolThis is one of the most important classes in JavaAssist, acting as a container for class data. ClassPool is responsible for managing CtClass objects, with each CtClass object representing a Java class. ClassPool provides methods to read and edit these classes.
2. CtClassCtClass is an abbreviation for 'compile-time class', representing a Java class. It provides interfaces for editing classes, such as adding fields, methods, constructors, etc. Once a CtClass object is frozen (i.e., after calling the toClass() method), it cannot be modified anymore.
3. CtMethod and CtFieldThese classes represent methods and fields within a class, allowing developers to access and set the code of methods or the properties of fields.
4. CtNewMethod and CtNewConstructor: These utility classes are used to quickly create new methods and constructors.
Implementation Method
JavaAssist modifies classes by manipulating bytecode, and its basic process is as follows:
1. Read or Create Class: Obtain or create a CtClass object through ClassPool.
2.Modify Class Structure: Modify the structure of the class by adding, modifying, or deleting CtField and CtMethod, etc.
3. Apply Changes: Call the `toClass()` method of CtClass to load the modified class into the JVM, or call `writeFile()` to write it to a file.
Example:
Dynamic Proxy Implementation
Assuming we need to create a dynamic proxy for an interface and add log output before and after each method call, we can use JavaAssist to achieve this:
import javassist.*; import java.lang.reflect.Method; public class DynamicProxy { public static Object createProxy(Class<?> interfaceClass) throws Exception { ClassPool pool = ClassPool.getDefault(); CtClass cc = pool.makeClass(interfaceClass.getName() + "Impl"); // Add interface cc.addInterface(pool.get(interfaceClass.getName())); // Add implementation for each method in the interface for (Method method : interfaceClass.getMethods()) { CtMethod cm = new CtMethod(pool.get(method.getReturnType().getName()), method.getName(), toCtClass(pool, method.getParameterTypes()), cc); StringBuilder methodBody = new StringBuilder("{\n"); methodBody.append("System.out.println("Before method " + method.getName() + "");\n"); methodBody.append("System.out.println(\"After method " + method.getName() + "\");\n") // Add return statement based on return type if (!method.getReturnType().equals(Void.TYPE)) { methodBody.append("return "); appendDefaultValue(methodBody, method.getReturnType()); methodBody.append(";\n"); } methodBody.append("}"); cm.setBody(methodBody.toString()); cc.addMethod(cm); } return cc.toClass().newInstance(); } private static CtClass[] toCtClass(ClassPool pool, Class<?>[] classes) throws NotFoundException { CtClass[] ctClasses = new CtClass[classes.length]; for (int i = 0; i < classes.length; i++) { ctClasses[i] = pool.get(classes[i].getName()); } return ctClasses; } private static void appendDefaultValue(StringBuilder builder, Class<?> type) { if (type.isPrimitive()) { if (type == Boolean.TYPE) { builder.append("false"); } else { builder.append("0"); } } else { builder.append("null"); } } }
This example demonstrates how to dynamically create a class that implements a specified interface using JavaAssist, and adds simple log output statements in each method. This technique can be applied to various scenarios such as AOP frameworks and simulation test objects.
2.3 Concurrency Containers and Utility Classes in Java
JAVA Security | In-depth analysis of the underlying mechanism of Runtime.exec command execution
After a period of stable operation of the online JAVA application, a JVM crash problem occurred
Four. Java Web Application Server (such as Tomcat)
Database入门:Master the five basic operations of MySQL database and easily navigate the data world!

评论已关闭