The concept and background of memory horses
Origin and development of memory horses
Memory horses originated as a technical means to circumvent traditional security detection, derived from the development of webshells. Early webshells mainly resided on servers by uploading malicious files, but with the advancement of file detection technology, this method has gradually been phased out, and memory horses have become a new attack method. It directly loads malicious code into the system memory, avoiding landing in the file system, making it difficult for traditional antivirus software and intrusion detection systems to identify.
Memory horses in the current network environment
With the increasing complexity of modern network environments, especially with the popularity of cloud computing and microservice architecture, the application scenarios of memory horses are expanding. The elasticity and dynamics of cloud computing make traditional protection methods such as file monitoring and static analysis no longer effective, and the concealment of memory horses is particularly prominent in such environments. Especially in containerized applications, memory horses can directly attack running containers without touching the file system, making them more difficult to detect.
Definition and classification of memory horses
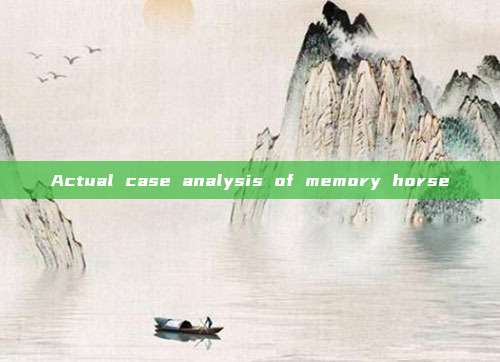
Memory horses are an attack method that runs malicious code directly in the system memory, mainly divided into the following categories:
- Memory horses based on the Servlet specification: By exploiting the Servlet API, attackers can dynamically register malicious Servlets or Filters, bypassing conventional request filtering and processing.
- Memory horses based on third-party components: In frameworks such as SpringMVC and Struts2, attackers inject malicious code through vulnerabilities to implant memory horses in the system.
- Memory horses based on Java Agent: By using the JVM's Instrumentation interface, attackers can modify runtime bytecode to achieve the purpose of memory injection.
Technical implementation of memory horses
Memory horse implantation and protection under the Servlet specification
In memory horses based on Servlets, attackers typically inject malicious code into the request handling chain of web applications by dynamically registering Filters or Servlets. For example, attackers can inject a malicious Filter into the Tomcat server using the following code:
// Example code for dynamically registering a malicious Filter public class MaliciousFilter implements Filter { @Override public void init(FilterConfig filterConfig) throws ServletException { // Initialization logic } @Override public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain) throws IOException, ServletException { // Malicious logic, such as stealing request data, recording user information, etc. // Continue to execute other filters or target resources chain.doFilter(request, response); } @Override public void destroy() { // Destruction logic } } // Dynamically register Filter in ServletContext FilterRegistration.Dynamic filterRegistration = servletContext.addFilter("maliciousFilter", new MaliciousFilter()); filterRegistration.addMappingForUrlPatterns(EnumSet.of(DispatcherType.REQUEST), false, "/*");
The above code demonstrates how to ServletContext Dynamically register a malicious Filter. This Filter can intercept all HTTP requests and execute specific malicious logic, such as stealing users' sensitive data or executing unauthorized commands.
Preventive measures:
- Code review and security testing: In the development and deployment stages, conduct strict code review and security testing to prevent unsafe dynamic registration code from entering the production environment.
- Use advanced protection tools: Deploy security tools that can detect and prevent unsafe dynamic behaviors, monitor and review the registration process of Servlets.
Memory horse attack path in third-party components
Taking the SpringMVC framework as an example, an attacker can control the application's HTTP requests by dynamically registering a malicious Controller.
// Example code for dynamically registering a malicious Controller @Controller public class MaliciousController { @RequestMapping("/malicious") public void handleRequest(HttpServletRequest request, HttpServletResponse response) throws IOException { // Malicious logic: such as reading request parameters and sending them to the attacker's server String data = request.getParameter("data"); sendToAttackServer(data); response.getWriter().write("Request handled by malicious controller."); } private void sendToAttackServer(String data) { // Simulate sending data to the attacker's server System.out.println("Sending data to attack server: " + data); } } // Dynamically register Controller RequestMappingHandlerMapping handlerMapping = applicationContext.getBean(RequestMappingHandlerMapping.class); Method method = MaliciousController.class.getMethod("handleRequest", HttpServletRequest.class, HttpServletResponse.class); HandlerMethod handlerMethod = new HandlerMethod(new MaliciousController(), method); RequestMappingInfo requestMappingInfo = RequestMappingInfo.paths("/malicious").build(); handlerMapping.registerMapping(requestMappingInfo, handlerMethod);
In this example, the malicious ControllerIt can be dynamically registered at runtime and can handle specific path HTTP requests. This attack method is extremely stealthy because it does not require modification of existing code; it can be completed simply through dynamic registration at runtime.
Preventive measures:
Security configuration: Ensure that the security configuration of third-party components is in place, and limit the abuse of dynamic registration features.
- Regular security audit: Conduct regular security audits of the application, especially for parts using third-party frameworks, to prevent potential security vulnerabilities.
Advanced attack and defense strategies of Java Agent
Java Agent allows modification of bytecode at JVM startup or runtime, which is an important approach for memory horse technology. The following is an example code for injecting a memory horse through Java Agent:
// Example code for Java Agent implementation of memory horse injection public class MaliciousAgent { public static void premain(String agentArgs, Instrumentation inst) { inst.addTransformer(new ClassFileTransformer() { @Override public byte[] transform(ClassLoader loader, String className, Class<?> classBeingRedefined, ProtectionDomain protectionDomain, byte[] classfileBuffer) throws IllegalClassFormatException { // Modify bytecode here if ("javax/servlet/http/HttpServlet".equals(className)) { // Insert malicious bytecode return injectMaliciousCode(classfileBuffer); } return classfileBuffer; } }); } private static byte[] injectMaliciousCode(byte[] classfileBuffer) { // Use ASM or Javassist to modify bytecode and add malicious logic // ... return modifiedClassBuffer; } }
In this example, the Java Agent is used at JVM startup through premainmethod registration of ClassFileTransformer,which dynamically modifies specific classes (such as HttpServlet)byte code, inject malicious logic. This method allows memory horses to be implanted without touching the original code, and has strong concealment.
- Memory forensics and real-time monitoring:Use memory forensics tools such as Volatility to analyze activities in memory, while deploying real-time monitoring systems to detect abnormal behaviors of bytecode modifications.
- Strict access control:Restrict the use permissions of Java Agent to ensure that only authorized operations can load and run Agent.
Memory horse detection and forensics
Technical challenges of memory horse detection
The fileless characteristic of memory horses makes it impossible for traditional detection methods to effectively discover their existence. Attackers often use obfuscation and encryption techniques to further increase the difficulty of detection. In the face of these challenges, the strategies for detecting memory horses include:
- Abnormal behavior analysis:By monitoring abnormal behaviors in memory, such as abnormal memory allocation and release operations, the activities of memory horses can be identified.
- Multi-level detection:Combining network traffic analysis, behavior monitoring, and memory forensics tools to form a multi-level detection system, improving the identification rate of memory horses.
Detection method based on behavior analysis
Behavior analysis technology, by monitoring the behavior patterns of the system, can effectively identify the activities of memory horses. For example, by monitoring the memory usage patterns of the system, abnormal activities of memory horses can be found. The following is a simple example code for memory usage pattern monitoring:
// Example code for memory usage pattern analysis public class MemoryUsageAnalyzer { private static final long MEMORY_THRESHOLD = 100 * 1024 * 1024; // 100 MB public void analyzeMemoryUsage() { Runtime runtime = Runtime.getRuntime(); long usedMemory = runtime.totalMemory() - runtime.freeMemory(); if (usedMemory > MEMORY_THRESHOLD) { System.out.println("Warning: High memory usage detected!"); // Execute further memory analysis operations, such as memory dump analysis } } }
This code monitors JVM memory usage and triggers an alert when memory usage exceeds the preset threshold.This method can help discover abnormal memory behavior of memory horses.
Application of memory forensics technology and tools
Memory forensics tools are one of the important tools for detecting memory horses. Through these tools, security experts can deeply analyze activities in memory and extract malicious code. The following are the basic steps for memory analysis using Volatility:
1、Obtain memory imageUse DumpIt and other tools to obtain the memory image of the target system.
2、Load image and analyzeUse Volatility to load memory images and use commands such as pslist, malfind, etc., to analyze processes and potential malicious activities in the system.
3、Extract malicious codeThrough analysis, extract malicious code from suspicious processes or memory areas for further reverse analysis.
4、Obtain memory imageUse tools (such as DumpIt) to obtain the memory image of the target system. This image will contain all memory data of the system at runtime, including processes, network connections, and memory horses.
Memory horse threats in the enterprise environment
Memory horses pose a serious threat to key business systems in the enterprise environment. The following is a case analysis of an enterprise memory horse attack:An ERP system of a manufacturing enterprise was attacked by a memory horse, causing the operation of the production line to be interrupted, resulting in tens of millions of economic losses.
Technical analysis:
- Attack methods:Attackers exploited the unpatched vulnerabilities existing in the ERP system, successfully planted memory horses, controlled the system remotely through malicious code in memory, and ultimately led to system paralysis.
- Detection and response:Due to the fileless nature of memory horses, traditional security measures of enterprises have failed to detect attacks in time, leading to attackers lurking in the system for several weeks.
Protection recommendations:
- Regular vulnerability scanning and repair:Ensure that all business systems are regularly scanned for vulnerabilities and security vulnerabilities found are repaired in a timely manner.
- Strengthen system logging and behavior monitoring:Through detailed logging and behavior monitoring, detect and respond to abnormal behaviors in a timely manner.
Memory horse handling in emergency response
In the emergency response process, the detection and removal of memory horses are critical steps. The following is a practical guide to emergency response operations:
1、Initial detection:Use behavior monitoring and memory forensics tools to identify suspicious memory activities.
2、Isolation and control:Once the existence of the memory horse is confirmed, immediately isolate the infected system to prevent the spread of the attack.
3、Cleaning and restoration:Use tools such as Volatility to extract and analyze malicious code, determine the cleaning strategy, and restore the system to normal operation.
4、Post-event analysis:After clearing the memory horse, conduct in-depth analysis of the attack path, summarize the lessons learned, and improve security protection measures.
Frontier technology of memory horse protection
Latest technology for enhancing memory protection
In the face of the continuous evolution of memory horse technology, the latest technology for enhancing memory protection is gradually developing. These technologies include memory sandboxes, memory integrity protection, and are aimed at limiting the execution capabilities of malicious code in memory. The following is an example of a basic memory sandbox implementation:
// Basic memory sandbox implementation public class MemorySandbox { private static final Set<String> ALLOWED_CLASSES = Set.of("com.example.SafeClass"); public static void execute(Runnable task) { String className = task.getClass().getName(); if (ALLOWED_CLASSES.contains(className)) { task.run(); } else { throw new SecurityException("Unauthorized class execution: " + className); } } }
In this example, the memory sandbox restricts only permitted classes to execute, and other classes that attempt to run will be thrown an exception.This method can effectively prevent the implantation and execution of memory horses.
The architecture of the automated defense systemConstruct
Building an automated defense system is an effective way to deal with memory horse attacks. The following is the basic architecture of an automated defense system:
- Real-time Monitoring Module: Monitor memory and system behavior, and issue timely alarms upon detecting anomalies.
- Response Execution Module: Automatically execute isolation, blocking, cleaning, and other operations based on predefined strategies.
- Analysis and Learning ModuleBy analyzing historical attack data, continuously optimizing defense strategies, and enhancing the system's defense capabilities.
The following is a simplified example of an automated defense system architecture code:
// A simplified example of an automated defense system architecture code public class AutomatedDefenseSystem { private final MemoryMonitor memoryMonitor; private final AnomalyDetector anomalyDetector; private final ResponseHandler responseHandler; public AutomatedDefenseSystem() { this.memoryMonitor = new MemoryMonitor(); this.anomalyDetector = new AnomalyDetector(); this.responseHandler = new ResponseHandler(); } public void startDefense() { memoryMonitor.startMonitoring(); memoryMonitor.onAnomalyDetected(data -> { boolean isMalicious = anomalyDetector.analyze(data); if (isMalicious) { responseHandler.executeResponse(data); } }); } }
In this example,MemoryMonitorThe module is responsible for monitoring system memory,AnomalyDetectorThe module analyzes monitoring data and detects anomalies while ResponseHandlerThe module takes automatic response measures when it detects anomalies. This architecture can be expanded into a more complex system, combining multiple detection and response mechanisms to provide comprehensive protection against memory horse attacks.
Future memory security challenges
In the future, as attackers continuously explore new memory attack methods, memory horse protection will also face more challenges. Potential new attack methods may include the use of AI to generate malicious code, memory attacks on IoT devices, etc. Therefore, security experts and enterprises must:
- Maintain forward-lookingContinuously track new technologies and new threats in the field of memory security and formulate protection strategies in advance.
- Strengthen cross-enterprise cooperationEstablish a cross-enterprise, cross-industry collaborative protection system to jointly respond to new memory threats.
Memory horse defense system construction
Building a comprehensive memory horse defense system
Building an effective memory horse defense system requires comprehensive design at the strategic, tactical, and operational levels. The following is a construction idea for a memory horse defense system:
- Strategic levelClarify the core goals of memory security, formulate security policies and budgets.
- Tactical levelDeploy multi-level security technologies such as memory sandboxes, behavior monitoring, and memory forensics tools.
- Operation levelImplement strict security operation and emergency response measures to ensure the effectiveness of the protection system.
Security governance and memory horse management
Security governance occupies an important position in the memory horse defense system. The following are some key measures of security governance:
- Memory security policy formulationDevelop clear memory security strategies to standardize memory usage and access control.
- Security audit and evaluationRegularly audit and evaluate the memory security measures to ensure their effectiveness.
- Event reporting and emergency responseEstablish a comprehensive event reporting mechanism to respond quickly when memory horse attacks are detected.
Combination of technology and management
In memory horse defense, the combination of technical protection and management measures is particularly important. While improving memory security through technical means, it is also necessary to ensure that these technologies can be effectively implemented through management measures. Enterprises can achieve the combination of technology and management through the following approaches:
- Safety culture constructionEnhance the safety awareness of employees to ensure that each employee is aware of the importance of memory security.
- Process standardizationEstablish standardized security operation procedures to ensure that protective measures are strictly implemented at each stage.
- Continuous improvementBased on the actual protective effect, continuously improve and optimize the protective measures to form a dynamic memory security protection system.

评论已关闭