Preface
Accessing a certain APP will lead to the APP download page, from which the target APP can be downloaded for analysis.
Install frida and upload frida-server
# frida-16.6.6-cp37-abi3-win_amd64
pip install frida
pip install frida-tools
pip install Pyro4
Download frida-server, which needs to be consistent with the frida version installed with your Python, and you need to check the emulator architecture
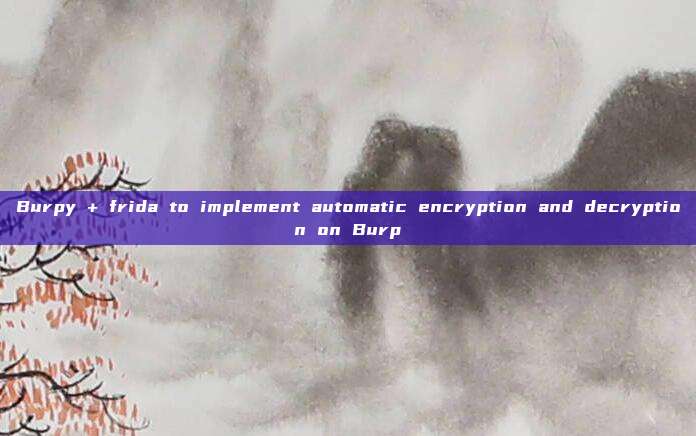
adb shell getprop ro.product.cpu.abi # View the bit number
Upload frida-server (if not connected to the device, remember to adb connect to the device first, this will not be elaborated here)
adb push frida-server /data/local/tmp/
Start frida-server, (frida-server is renamed by me)
adb root
adb shell
cd /data/local/tmp
chmod +x frida-server
https://www.freebuf.com/articles/es/frida-server
Start another cmd to view the emulator process to verify whether frida is connected successfully: a process-related information means a successful connection.
frida practical test
Packet capture shows that all data packets are encrypted content, how to deal with this, the broadcast is over!:
First, check the running processes and package name information
frida-ps -Ua
Here, the package name of my program is: calm.pjtuep.zzdokmht
Next, write the hook script to output the strings and related call stack information that appear during the data interaction of the APP:
Java.perform(function () {
var StringCls = Java.use("java.lang.String"); // Get the Java String class
// Hook the getBytes() method (no parameter version)
StringCls.getBytes.overload().implementation = function () {
var result = this.getBytes(); // Call the original getBytes() method
// Filter out short strings and only record strings longer than 16 characters
if (this.length() > 16) {
// Get the call stack information
console.log("[*] Stack trace:==========>\n" +
Java.use("android.util.Log").getStackTraceString(
Java.use("java.lang.Exception").$new()
)
);
console.log("[*] getBytes() called with ==============>: " + this); // Output the current string
}
return result; // Return the original result to ensure that it does not affect normal execution
});
});
Why hook the getBytes method and code explanation:
frida -U -f "calm.pjtuep.zzdokmht" -l hook_key.js # Using the -f parameter will reload the APP, load the hook script, and you can enter exit by pressing Enter to exit.
You can see that the encrypted data in the response packet is hooked by the hook script and the call stack information is printed out, among which the most suspicious and obvious file is: ApiEncryptUtil.java
Therefore, use jadx-gui to decompile the apk and find ApiEncryptUtil.java
Code analysis: AES encryption and decryption, a is the decryption function, b is the encryption function
public static String a(String str) {
try {
byte[] decode = Base64.decode(str, 0); // Decode Base64 first
byte[] bytes = "0nxG8fD2kqlrEv5M".getBytes(); // AES key
byte[] bytes2 = "u0r3GcsdXsYmAfhT".getBytes(); // AES IV
SecretKeySpec secretKeySpec = new SecretKeySpec(bytes, "AES"); // Generate key
IvParameterSpec ivParameterSpec = new IvParameterSpec(bytes2); // Generate IV
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); // Use AES-CBC
cipher.init(2, secretKeySpec, ivParameterSpec); // 2 = decryption mode
return new String(cipher.doFinal(decode), C.UTF8_NAME); // Decrypt and convert back to string
} catch (Exception e2) {
e2.printStackTrace();
return "";
}
}
public static String b(String str) {
try {
byte[] bytes = str.getBytes(); // Obtain the byte array of the string
byte[] bytes2 = "0nxG8fD2kqlrEv5M".getBytes(C.UTF8_NAME); // AES key
byte[] bytes3 = "u0r3GcsdXsYmAfhT".getBytes(C.UTF8_NAME); // AES IV
SecretKeySpec secretKeySpec = new SecretKeySpec(bytes2, "AES"); // Generate key
IvParameterSpec ivParameterSpec = new IvParameterSpec(bytes3); // Generate IV
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding"); // Use AES-CBC
cipher.init(1, secretKeySpec, ivParameterSpec); // 1 = encryption mode
return new String(Base64.encode(cipher.doFinal(bytes), 0)); // Base64 encode after encryption
} catch (Exception e2) {
e2.printStackTrace();
return "";
}
}
Write a hook script to try to output encrypted and decrypted data:
Java.perform(function(){
var targetClass = Java.use('c.h.a.m.r'); // The package path should be filled according to the decompilation result on your computer
// Call the a decryption function and output the content
targetClass.a.implementation = function(str){
console.log("Decrypted data before ======>: "+ str + "\n\n\n");
var result = this.a(str);
console.log('Decrypted data after ======>: '+ result + "\n\n\n");
return result;
}
// Call the b encryption function and output the content
targetClass.b.implementation = function(str){
console.log("Encrypted data before ======>: "+ str + "\n\n\n");
var result = this.b(str);
console.log('Encrypted data ======>: '+ result + "\n\n\n");
return result;
}
});
The entire data transmission process:
In this way, we can see the decrypted and encrypted data, but it is still too麻烦 to test in burp, so we still need to use a simpler method.
Burpy + frida to implement automatic encryption and decryption on Burp
Next, use the Burpy plugin to implement automatic encryption and decryption on Burp, which is convenient for us to test.
View the device name, obtain the device name for script writing convenience
My mumu emulator device is on 127.0.0.1:16384, so this is the device: Device(id="127.0.0.1:16384", name="PGBM10", type=’usb’), which will be used for script writing later
>>> import frida
>>> frida.get_device_manager().enumerate_devices()
[Device(id="local", name="Local System", type='local'), Device(id="socket", name="Local Socket", type='remote'), Device(id="barebone", name="GDB Remote Stub", type='remote'), Device(id="127.0.0.1:16384", name="PGBM10", type='usb')]
Set port forwarding
adb forward tcp:27043 tcp:27043
adb forward tcp:27042 tcp:27042
# View connection status
adb forward --list
# Help
adb --help | findstr "forward"
Enter the emulator to start frida-server
adb root
adb shell
ali:/data/local/tmp # https://www.freebuf.com/articles/es/frida-server
Edit the rpc hook script to facilitate the calling of encryption and decryption methods on the APP for encryption and decryption:
The name of my file is: decrypt1.js
Java.perform(function () {
var targetClass = Java.use('c.h.a.m.r');
rpc.exports = {}}}
init: function () {
console.log("[Frida] rpc.exports initialization successful!");
return "rpc.exports has been loaded";
},
enc: function (str) {
try {
console.log("************ Start encryption ***********");
console.log("Encrypted data before: " + str);
var result = targetClass.b(str);
console.log("Encrypted data: " + result);
return result || ""; // **Prevent returning null**
} catch (e) {
console.log("[Frida] enc method execution error: " + e);
return ""; // **Prevent freezing**
}
},
dec: function (str) {
try {
console.log("************ Start decryption ***********");
console.log("Encrypted data before: " + str);
var result = targetClass.a(str);
console.log("Decrypted data: " + result);
return result || ""; // **Prevent returning null**
} catch (e) {
console.log("[Frida] dec method execution error: " + e);
return ""; // **Prevent freezing**
}
}
});
});
burpy.py script writing:
import frida
import time
from urllib.parse import unquote, parse_qs, quote
import json
class Burpy:
# Initialization, get the emulator process, start and connect the process
def __init__(self):
device = self._get_android_usb_device()
pid = device.spawn("calm.pjtuep.zzdokmht") # Process package name
self.session = device.attach(pid) # Attach to the target process
device.resume(pid) # Resume the process
self.rpc = self.load_rpc() # 加载 RPC 脚本
# 获取模拟器设备
def _get_android_usb_device(self):
for x in frida.get_device_manager().enumerate_devices():
if "PGBM10" in x.name: # 根据设备名,返回设备相关信息。
print(f"设备信息=====> {x}")
return x
# 加载远程调用rpc的hook脚本文件
def load_rpc(self):
# 这里打开的文件路径貌似只能写绝对路径,用相对路径会报错?
with open("D:\\secTools\\BurpSuite V2023.2.2\\BurpSuite V2023.2.2\\Burpy\\js\\decrypt1.js",encoding="utf-8", errors="ignore") as f:
script = self.session.create_script(f.read())
script.load()
self.rpc = script.exports_sync
time.sleep(3) # 等待APP加载完全
self.rpc.init() # 检测rpc调用
return self.rpc
# 对body数据处理,只返回data字段的数据
def convert_to_json(self, body):
try:
if body.strip().startswith('{') and body.strip().endswith('}'):
json_body = json.loads(body)
if 'data' in json_body:
return json_body['data']
else:
parsed_data = parse_qs(body)
return parsed_data['data'][0]
except Exception as e:
print(f"Conversion failed: {str(e)}")
return None
# 重新构建请求和响应body,方便发包和查看响应
def rebuild_body(self, body, data):
try:
if body.strip().startswith('{') and body.strip().endswith('}'):
json_body = json.loads(body)
if 'data' in json_body:
json_body['data'] = data
# print(str(json_body).encode("gbk").decode("gbk"))
return str(json_body).encode("gbk").decode("gbk") # Chinese garbled text occurred in the response content of the repeater, not clear why?
else:
parsed_data = parse_qs(body)
string_body = "timestamp=" + str(parsed_data['timestamp'][0]) + "&" + "data=" + data + "&" + "sign=" + str(parsed_data['sign'][0])
return string_body
except Exception as e:
print(f"Conversion failed: {str(e)}")
return None
# Encrypt function
def encrypt(self, header, body):
try:
process_data = self.convert_to_json(body)
enc_data = self.rpc.enc(process_data)
body = self.rebuild_body(body, enc_data)
except Exception as e:
print(f"Cannot find enc method: {str(e)}")
return header, body
# Decrypt function
def decrypt(self, header, body):
try:
process_data = self.convert_to_json(body)
dec_data = self.rpc.dec(process_data)
body = self.rebuild_body(body, dec_data)
except Exception as e:
print(f"Cannot find dec method: {str(e)}")
return header, body
Here is the writing of the burpy.py script, which needs to be written according to the actual situation. The process package name and the code to obtain the simulator device should be written according to the information you have viewed. The code is the same, because in the current package captured, it is found that there is also a timestamp and sign in the request package, and the response package is a json format data, which also contains other parameters. Analysis shows that only the data field is encrypted in both requests and responses, so when sending data packets and decrypting response packets, it is necessary to perform parameter splicing and encryption/decryption processing on the request body and response body, so that an automatic encryption and decryption request and response can be completed smoothly. The specific details can be known from the code analysis.
Set burpy:
Checking the box for enable processor can make it convenient to use in the Intruder爆破 module.
After filling in the Python path and burpy.py path, and writing the burpy.py and hook script, and confirming that there are no errors, you can click the Start server button. At this time, the simulator will automatically open the APP, and the hook script and Python script will also start running, and some output can be seen in the console (if you have written output log code.).
At this time, in the history record, right-click -> expand -> Burpy -> select decrypt function will pop up a window with the decrypted content:
The console will also output the content, because I wrote the corresponding code to show the decryption process.
Send the data packet to the repeater, first right-click to decrypt the request packet content, then click send to achieve automatic encryption and decryption:
Console log:
Send after modifying the package:
It is also normal.
Then if you want to debug your code, after modifying burpy.py, you can click Reload Script to reload the code, at this time the simulator will reconnect and open the APP again.
Some strange problems
When using the Burpy plugin, I found that: once the Python script runs into an error, Burp will freeze and go white, at this time you can only close Burp, open a new one, and then configure the proxy again, which feels a bit麻烦, and there is no response when clicking select file, don't know what the situation is, the path and other things are filled in manually… …
Chinese characters are garbled in the response content in the repeater, while the window opened by the burpy plugin does not have garbled characters :(
Some concept explanations
What is Frida?
Frida is a hook framework based on Python + JavaScript, which is essentially a dynamic instrumentation technology. It can be used on various platforms such as Android, Windows, and iOS, with its execution scripts written in Python or Node.js, and the injected code written in JavaScript. To put it simply, there is a tool called Frida, which can inject some JavaScript code into the process of executing an APP (taking APP as an example) to facilitate debugging and viewing the internal situation of the APP, such as data, called functions, etc., or modify some parameters in the process of APP running, such as intercepting the gold value in the APP and changing it to the desired gold value, etc.
What is hook technology?
Hook is essentially adynamic code injectionorfunction hijackingtechnology, which allows developers or attackers to intercept, modify, or replace the behavior of functions during program execution. For example:
intercepting system API calls(such as
send
,recv
,open
,read
)Modifying application logic(such as tampering with in-game gold, health)
Monitoring application behavior(such as capturing keyboard input, obtaining application memory data)
In fact, it is somewhat like a man-in-the-middle attack, such as during the APP running process, through hook technology to listen to or tamper with some events, such as player A attacks a wild monster, the wild monster's health decreases, we can use hook technology to hook out this event, view player health/wild monster health/how much attack power this player's sword attack, etc. (monitoring application behavior); and we can use hook technology to modify the attack power of the player's sword, achieve 999 attacks with one sword, etc. (modifying application logic); and we can view which function method player A specifically called for this sword (intercept/inspect system API calls).
Then Frida hook is easy to understand, it is to hook with Frida.
Why is adb forward port forwarding necessary?
adb forward tcp:27042 tcp:27042
and similar commands are used to map the port (such as 27042) on the Android/emulator device to the corresponding port on the host. This is important forfrida
It is necessary for Frida to communicate through network ports, especially when it is running on a remote device or emulator.
Explanation:
adb forward
commandThis command forwards the port on the device to the port on the host. It creates a port mapping path through the ADB connection.
27042
and27043
port:
frida-server
It will start and listen on a specific port on the Android device (usually 27042), which is used to communicate withfrida
client to communicate.port
27043
It is also often used for additional connections, especially when interacting between multiple processes or services.
Why is port forwarding necessary?
andfrida-server
communicationFrida client needs to communicate withfrida-server
Communication usually occurs through TCP/IP ports.adb forward
The command allows the Frida client on the host to communicate with the Android device by forwarding the port to the corresponding port on the host.frida-server
communicate.
remote communication: Whenfrida-server
When running on the device, it listens to the device's ports (such as 27042).adb forward
Allows you to access this port from the host, just like directly connecting to the device. In this way, you can control processes on the device, perform hook operations, and debug, etc.
The necessity of ports:
frida-server
Run on Android devices and listen to port 27042.frida
The client communicates with the device through this port. Without port forwarding, the host cannot access the device'sfrida-server
, which leads to the inability to perform hook operations or carry out other debugging tasks on the device.
Summary: Forwarding ports is to facilitate communication between the frida client on the host and the frida-server/frida server on the Android/simulator, making it convenient for us to debug/hook/ connect to processes on the device (such as APP).
Reference
frida-server download and installation:https://blog.csdn.net/yongmonkey/article/details/124123736
frida:https://github.com/frida/frida/releases
https://cloud.tencent.com/developer/article/2348148
jadx-gui download:https://github.com/skylot/jadx/releases
Burpy download:https://github.com/mr-m0nst3r/Burpy/releases
https://mp.weixin.qq.com/s/Fwq96_VKIduXAbrPp8kshw
https://github.com/hookmaster/frida-all-in-one
https://sunny250.github.io/2021/02/14/hook%E7%A5%9E%E5%99%A8FRIDA/
https://www.kancloud.cn/alex_wsc/android/506821
https://blog.csdn.net/weixin_39190897/article/details/115354102
Author of this article: Track-I am Big White
More SRC practical content, practical experience sharing, penetration techniques, vulnerability挖掘&vulnerability certificate acquisition techniques, follow me, subscribe to more exciting content~

评论已关闭