0x00. Overview
In Windows systems, some directories or files have improper permission settings, which allow attackers to plant malicious files or execute files under these directories. Due to the lack of effective access control and security review in these directories, attackers can exploit vulnerabilities to modify, replace, or inject files, even hijack legitimate processes or services in the system.
In Windows systems, there are some typical weak permission directories, such asC:\Windows\Temp
,C:\ProgramData
such as, these directories are usually used to store temporary files. However, many applications and users do not set sufficient permission controls for these directories when using them. Attackers can achieve file hijacking attacks by placing malicious executable files in these directories, thereby executing code or elevating system privileges.
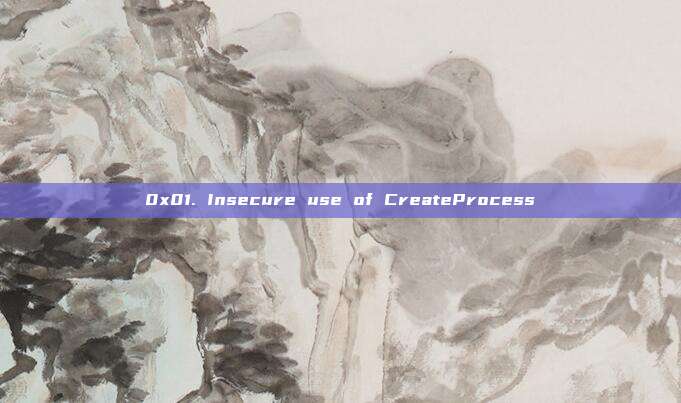
This article shares several file hijacking cases to understand the security issues brought by weak permission directories. Before discussing specific cases, let's talk about the CreateProcess API first.
0x01. Insecure use of CreateProcess
CreateProcess
API is the basic function used in Windows to create new processes, and its mechanism is crucial for the program's startup and path resolution. This API has multiple parameters, includinglpApplicationName
and}}lpCommandLine
are key parameters that collectively influence the behavior of process creation, especially how to parse and execute the executable file path passed in.
CreateProcess
The basic usage of
CreateProcess
The prototype of
BOOL CreateProcess(
LPCWSTR lpApplicationName, // Application name (optional)
LPWSTR lpCommandLine, // Command line arguments
LPSECURITY_ATTRIBUTES lpProcessAttributes, // Process security attributes
LPSECURITY_ATTRIBUTES lpThreadAttributes, // Thread security attributes
BOOL bInheritHandles, // Whether to inherit handles
DWORD dwCreationFlags, // Creation flags
LPVOID lpEnvironment, // Environment variables
LPCWSTR lpCurrentDirectory, // Current directory
LPSTARTUPINFO lpStartupInfo, // Startup information
LPPROCESS_INFORMATION lpProcessInformation // Process information
);
lpApplicationName
The path specified for the application (optional). IfNULL
then the system will parse fromlpCommandLine
the first space-separated item parses out the application path.lpCommandLine
The command line parameters passed to the new process. IflpApplicationName
isNULL
This parameter must include the complete path of the application or the command name.
lpApplicationName
isNULL
the path parsing when
whenlpApplicationName
isNULL
the system must parse fromlpCommandLine
This process involves path parsing and processing, which may involve file names containing spaces.
the order of path parsing in the command line
the CreateProcess documentation
Look at an example from a Microsoft official document, assuminglpCommandLine
it includes content similar to the following:
c:\program files\sub dir\program name
When CreateProcess executes the path without quotes, andlpApplicationName
isNULL
The system will parse the path in the following order:
c:\program.exe
The system will first try to truncate the path from the beginning of the string and parse it asc:\program.exe
.c:\program files\sub.exe
If the first parsing fails, the system will attempt to parse the path asc:\program files\sub.exe
.c:\program files\sub dir\program.exe
:Next, the system will try to parse the entire path, thinkingprogram.exe
is the executable file name, and try to execute it.c:\program files\sub dir\program name.exe
:Finally, the system will try toprogram name
as an executable file name and append.exe
extension for parsing.
Write poc program to test
Test program:
#include <windows.h>
#include <stdio.h>
#include <stdlib.h>
int main() {
// Define and initialize the command line
char *szCmdline = _strdup("c:\\program files\\sub dir\\program name");
// Initialize the STARTUPINFO and PROCESS_INFORMATION structures
STARTUPINFOA si = {0}; // Use ANSI structure
PROCESS_INFORMATION pi = {0}; // Process information
si.cb = sizeof(si);
// Call CreateProcessA (ANSI version)
if (CreateProcessA(
NULL, // Application name, NULL indicates to parse the path from the command line
szCmdline, // Quoted command line
NULL;
NULL;
FALSE;
0;
NULL;
NULL;
&si;
π
)) {
printf("Process created successfully!\n");
// Wait for the child process to complete
WaitForSingleObject(pi.hProcess, INFINITE);
// Close the handles
CloseHandle(pi.hProcess);
CloseHandle(pi.hThread);
} else {
printf("Failed to create process. Error code: %lu\n", GetLastError());
}
// Release the command line buffer
free(szCmdline);
return 0;
}
The test program attempts to start "c:\program files\sub dir\program name" through CreateProcessA, compile and run the program, and at the same time use Process Monitor to monitor
As can be seen, Process Monitor has monitored the expected behavior of the program. If program.exe exists at the root directory of the C drive, then c:\program.exe will be executed.
The safe usage of the CreateProcess API should be:
LPTSTR szCmdline[] = _tcsdup(TEXT("\"C:\\Program Files\\MyApp\" -L -S"));
CreateProcess(NULL, szCmdline, /*...*/);
If lpApplicationName is set to NULL, the executable file path in lpCommandLine must be quoted. There are similar behavior API functions like CreateProcessAsUser.
0x02. Directory Permissions and File Hijacking
Through the above CreateProcess test program, it can be seen that some irregular coding habits may cause unexpected behavior in the program, which has potential security risks. If the relevant directory is set to weak permissions, for example, if the c:\program files\sub dir\ directory permission settings are incorrect, an attacker with ordinary permissions can write a malicious file in this directory, and use file hijacking to achieve the purpose of privilege escalation. Next, let's explore the potential dangers of file hijacking caused by weak permissions directories through several real CVE cases.
0x03. Case Analysis
CVE-2020-13884: exe hijacking caused by weak permissions directory
InCVE-2020-13884During the Citrix program uninstallation process, the CreateProcess API is called to execute the file TrolleyExpress.exe (C:\ProgramData\Citrix\Citrix Workspace 1911\TrolleyExpress.exe). Due to the unquoted path, the program attempts to load C:\ProgramData\Citrix\Citrix.exe. The path C:\ProgramData\Citrix\has weak permissions, allowing an attacker to write a malicious Citrix.exe at this path. They wait for the administrator to uninstall the Citrix Workspace application, at which point the malicious Citrix.exe is executed to elevate privileges.
CVE-2022-24767: Local privilege escalation caused by weak permission system directory
CVE-2022-24767 Summary: When running under the SYSTEM user account, the uninstaller of Windows Git is vulnerable to DLL hijacking
The system user uninstalls the Git for Windows program, monitors the program behavior, and finds that the Git uninstaller will try to download fromC:\Windows\Temp
loading dll
Since ordinary users also haveC:\Windows\Temp
directory write permission, so low privilege attackers can write malicious dlls toC:\Windows\Temp
directory, when the system user uninstalls the Git program, the malicious dll will run, and the attacker can achieve the purpose of privilege escalation.
Here, an attempt is made to execute malicious code by hijacking netapi32.dll
Malicious netapi32.dll test code:
#include<stdio.h>
#include<windows.h>
BOOL WINAPI DllMain (HANDLE hDll, DWORD dwReason, LPVOID lpReserved){
if (dwReason == DLL_PROCESS_ATTACH){
system("cmd.exe \
ExitProcess(0);
}
return TRUE;
}
After compilation, place netapi32.dll in the C:\Windows\Temp directory, and when the system user uninstalls the Git for Windows program, it will be found that the malicious dll is executed, successfully adding a user hacker to the administrator group
CVE-2022-39845: Arbitrary directory deletion caused by weak permission directories
This vulnerability is about Samsung Kies (a PC suite tool provided by Samsung that can connect mobile phones to computers), where improper verification exists during program uninstallation, allowing local attackers to exploit directory links to delete any directory. Using Process Monitor to monitor the file uninstallation process, it was found that the program attempts to delete the contents of the C:\ProgramData\Samsung\deviceprofile\cache directory, while this directory does not exist.
The C:\ProgramData directory has weak permissions. Checking the directory permissions of C:\ProgramData\Samsung\, it can be seen that ordinary users can read and write.
A low-privileged attacker can create the C:\ProgramData\Samsung\deviceprofile\cache directory and link it to other high-privileged directories. Then, when the administrator user uninstalls the program, it can achieve the attack effect of deleting any directory.
For example, if an ordinary user tries to delete the contents of the C:\Windows\hacktest\ directory, they will find that they do not have permission.
However, ordinary users can create a directory symbolic link, linking C:\ProgramData\Samsung\deviceprofile\cache to C:\Windows\hacktest
In this way, when the administrator uninstalls the Samsung Kies program, it will cause the contents of the C:\Windows\hacktest\ directory to be deleted.
0x04. Summary
Program directory permission-related file hijacking is a frequently overlooked security issue, usually involving improper permission control, path configuration errors, or inadequate file system management. By strengthening file permission management, path security, and file integrity protection, it is possible to effectively prevent such attacks from occurring.
0x05. Reference link:
https://learn.microsoft.com/zh-cn/windows/win32/api/processthreadsapi/nf-processthreadsapi-createprocessa?redirectedfrom=MSDN
https://security.samsungmobile.com/serviceWeb.smsb?year=2022&month=09
https://www.freebuf.com/vuls/400438.html

评论已关闭