0x00 Preface
With the continuous updates and iterations of the Windows system, the technology of Windows driver development is also constantly upgraded: from the earliest VXD (Virtual X Driver) (which has been deprecated) to the WDM (Windows Driver Model) driver model introduced on Windows 2000, followed by the WDF (Windows Driver Foundation) driver model introduced on Windows Vista, which has been carried forward to the present; WDF is an upgraded version of WDM and is somewhat compatible, and WDF is the recommended driver development model by Microsoft at present.
WDF can be further divided into kernel-mode KMDF (Kernel-Mode Driver Framework) and user-mode UMDF (User-Mode Driver Framework), as the name implies, UMDF will have more restrictions in order to achieve higher operating system stability, and its binary extension name is*.dll
; The development of UMDF and KMDF is basically the same, and this article only introduces the development using the more widely used KMDF.
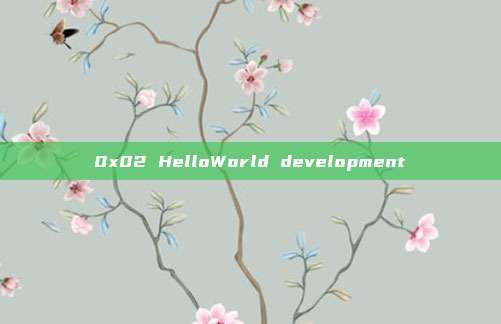
This article's experimental environment
Windows 10 Professional x64 1909
Visual Studio 2019
SDK 10.0.19041.685
WDK 10.0.19041.685
0x01 Set up the driver development environment
Firstly, configureVisual Studio 2019
C/C++ development environment (https://visualstudio.microsoft.com/), follow the official Visual Studio tutorial to automatically download and install 'Desktop Development with C++', where the SDK is set to default as10.0.19041.0
:
Development software requires SDK (Software Development Kit), and developing Windows drivers requires WDK (Windows Driver Kit); now let's configure the WDK environment, download the WDK online installer package from the official website (https://learn.microsoft.com/zh-cn/windows-hardware/drivers/download-the-wdk) (the version must be consistent with the SDK), and install it as follows:
For the WDF driver model, its development environment is called WDK (Windows Driver Kit)
For the WDM driver model, its development environment is called DDK (Driver Development Kit)
After installation is complete, the window will be checked by defaultVisual Studio
Install the WDK extension plugin and follow the guidance to install it. After that, we canVisual Studio
On the Create Project page, you will see options such as KMDF/UMDF, indicating that the Windows driver development environment has been successfully configured.
The Windows driver development environment may be affected by the version of the operating system, Visual Studio, SDK, and WDK. The configuration process requires careful attention to these aspects. If you encounter any problems, you can refer to the following version information: https://learn.microsoft.com/en-us/windows-hardware/drivers/other-wdk-downloads.
0x02 HelloWorld development
According to the official tutorial, we create a project inVisual Studio
Create an emptyKMDF
Create a projectDriver.c
Write the code as follows in the file
#include <ntddk.h>
#include <wdf.h>
DRIVER_INITIALIZE DriverEntry;
EVT_WDF_DRIVER_DEVICE_ADD KmdfHelloWorldEvtDeviceAdd;
NTSTATUS
DriverEntry(
_In_ PDRIVER_OBJECT DriverObject,
_In_ PUNICODE_STRING RegistryPath
)
{
// NTSTATUS variable to record success or failure
NTSTATUS status = STATUS_SUCCESS;
// Allocate the driver configuration object
WDF_DRIVER_CONFIG config;
// Print "Hello World" for DriverEntry
KdPrintEx((DPFLTR_IHVDRIVER_ID, DPFLTR_INFO_LEVEL, "KmdfHelloWorld: DriverEntry\n"));
// Initialize the driver configuration object to register the
// entry point for the EvtDeviceAdd callback, KmdfHelloWorldEvtDeviceAdd
WDF_DRIVER_CONFIG_INIT(&config,
KmdfHelloWorldEvtDeviceAdd
);
// Finally, create the driver object
status = WdfDriverCreate(DriverObject,
RegistryPath,
WDF_NO_OBJECT_ATTRIBUTES,
&config,
WDF_NO_HANDLE
);
return status;
}
NTSTATUS
KmdfHelloWorldEvtDeviceAdd(
_In_ WDFDRIVER Driver,
_Inout_ PWDFDEVICE_INIT DeviceInit
)
{
// We're not using the driver object,
// so we need to mark it as unreferenced
UNREFERENCED_PARAMETER(Driver);
NTSTATUS status;
// Allocate the device object
WDFDEVICE hDevice;
// Print "Hello World"
KdPrintEx((DPFLTR_IHVDRIVER_ID, DPFLTR_INFO_LEVEL, "KmdfHelloWorld: KmdfHelloWorldEvtDeviceAdd\n"));
// Create the device object
status = WdfDeviceCreate(&DeviceInit,
WDF_NO_OBJECT_ATTRIBUTES,
&hDevice;
);
return status;
}
Set it to in the 'Configuration Manager'Debug/x64
, when compiling and generating the project, the following error was found:
Visual Studio
The mechanism to mitigate Spectre attacks is enabled by default, and you can select and install specific support libraries through the VS Installer in our experimental environment, where we can directly disable this feature, inProject Properties - C/C++ - Code Generation - Spectre Mitigation
Set toDisable
:
After that, the compilation can be successfully completed, in[src]/x64/Debug/
Below, you can see the generated binary fileKmdfHelloWorld.sys
0x03 Deployment and Testing
The driver usually runs in the form of a service or device, which is different from a regular application, so it cannot be debugged and tested like an application. The official guidance provides a driver debugging method based on a dual-machine debugging environment, which is somewhat繁琐, and we will briefly introduce it here; those who want to learn about a more convenient local driver debugging method can jump to '0x04 Local Driver Debugging'.
According to the official guidance, we will run and debug the driver as a device, and before that, we need to provide another host as the debugged machine (debugee
), the driver will be debugged on the debugged machine (debugee
) for deployment and testing, and this host acts as both the development host and the debugging machine (debugger
), as follows:
First on the debugged machine (debugee
) also install the WDK environment, and then run the tool in the WDK installation directoryWDK Test Target Setup
), default path:C:\Program Files (x86)\Windows Kits\10\Remote\x64\WDK Test Target Setup x64-x64_en-us.msi
; After that, in the debug machine (debugger
) inVisual Studio
connect to the debugged machine (debugee
) inWDK Test Target Setup
) tool, automatically complete the configuration of the dual-machine debugging environment.
on the development host (debugger
) Initialize the relevant information of the debugged machine inProject Properties-Driver Install-Deployment
In which we add a new device, we use the network dual-machine debugging method here, with default parameters:
and the configuration will be automatically completed as follows:
) is being debuggedVisual Studio
in which the debugged machine (debugee
) After adding, select this host in the following window and set the driver's hardware ID toRoot\KmdfHelloWorld
, as follows:
After the configuration is complete, we can see inVisual Studio
menuGenerate-Deploy Solution
The driver will be automatically deployed on the debugged machine (debugee
) and run the test:
on the debugged machine (debugee
) and we can see it in the Device ManagerKmdfHelloWorld
Successfully deployed:
If you want to debug the driver, you can use WinDBG to debug the driver based on the dual-machine debugging environment mentioned above.
0x04 Debugging the driver on the local machine
The official driver deployment and testing methods, although effectively isolating the development environment and debugging environment, are indeed too cumbersome, not to mention the various problems under the dual-machine debugging environment. Moreover, the driver can also be run as a service, and through this method, we can debug the driver on the local machine more conveniently.
In daily security work, I prefer to use this method because most of the time I only need to work on kernel-level driver code, without concerning whether it is a complete Windows driver device. This method can help me quickly carry out security verification work.
Create a KMDF project and write the code as follows:
#include <ntddk.h>
#include <wdf.h>
VOID OnUnload(IN PDRIVER_OBJECT DriverObject)
{
UNREFERENCED_PARAMETER(DriverObject);
KdPrintEx((DPFLTR_IHVDRIVER_ID, DPFLTR_INFO_LEVEL, "test: unload driver\n"));
}
NTSTATUS
DriverEntry(
_In_ PDRIVER_OBJECT DriverObject,
_In_ PUNICODE_STRING RegistryPath
)
{
UNREFERENCED_PARAMETER(DriverObject);
UNREFERENCED_PARAMETER(RegistryPath);
KdPrintEx((DPFLTR_IHVDRIVER_ID, DPFLTR_INFO_LEVEL, "test: driver entry\n"));
DriverObject->DriverUnload = OnUnload;
return STATUS_SUCCESS;
}
Set it to in the 'Configuration Manager'Debug/x64
, compile and generate the project.
Before running the test, we need to enable test mode on the local machine (i.e., the development host) (the restart takes effect), so that the operating system can load the driver we compiled, open powershell with administrative privileges:
# Enable test mode
bcdedit /set testsigning on
After restarting the host, open powershell with administrative privileges throughsc.exe
The command creates a service for the driver (for detailed explanation, please refer to: https://learn.microsoft.com/en-us/windows-server/administration/windows-commands/sc-create):
# Create a service named test with type kernel and start type demand, and specify the driver program path
# (Note that there must be a space after the equal sign of the parameter)
sc.exe create test type= kernel start= demand binPath= C:\Users\john\Desktop\workspace\kmdf_test\x64\Debug\kmdf_test\kmdf_test.sys
# Use queryex to view the created service information
sc.exe queryex test
Create the service as follows:
Then you can usesc.exe
to start the driver program and useDebugView
View debugging output (checkCapture Kernel
andEnable Verbose Kernel Output
to see the output):
# Start the driver program
sc.exe start test
# Stop the driver program
sc.exe stop test
Run as follows:
After updating the driver code and compiling the project, you can do it again:start/stop
This service allows for quick testing and debugging of driver code.
0x05 References
https://learn.microsoft.com/zh-cn/windows-hardware/drivers/gettingstarted/
https://github.com/microsoft/Windows-driver-samples</https://github.com/microsoft/Windows-driver-samples>
https://visualstudio.microsoft.com/
https://learn.microsoft.com/zh-cn/windows-hardware/drivers/download-the-wdk</https://learn.microsoft.com/zh-cn/windows-hardware/drivers/download-the-wdk>
https://learn.microsoft.com/en-us/windows-server/administration/windows-commands/sc-create</https://learn.microsoft.com/en-us/windows-server/administration/windows-commands/sc-create>
Development of Burp plugin for sensitive information detection based on deepseek local large model
From Gartner's '2021 Security and Risk Management Trends' to the development of XDR
Is data security work too abstract? Share a business development approach (Part I: Thinking)
Cloud Native Security Series 1: Zero Trust Security and Software Development Life Cycle

评论已关闭